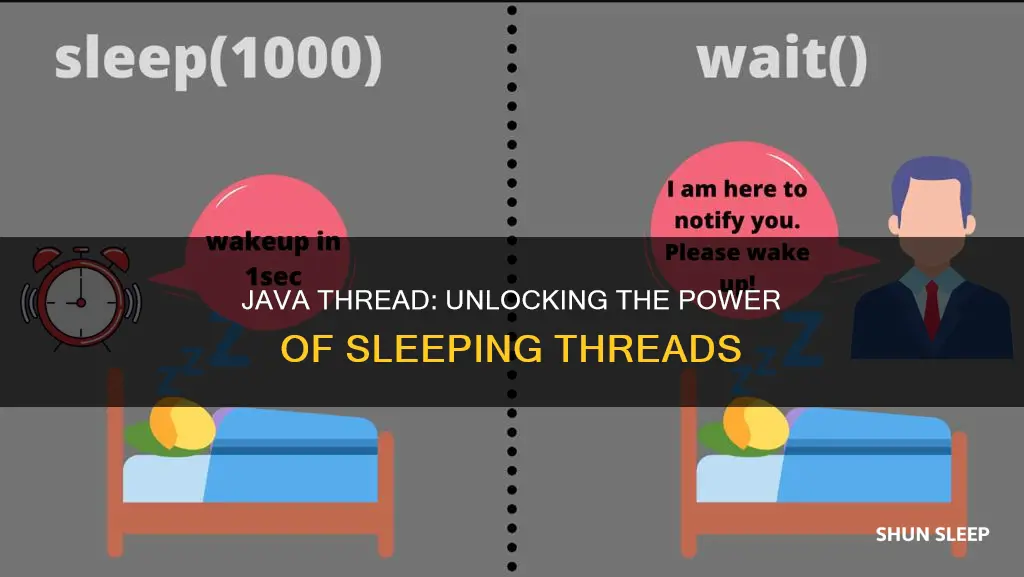
In Java, threads can enter a sleeping state using the `Thread.sleep()` method, which pauses the execution of a thread for a specified amount of time. However, sometimes it's necessary to wake up a sleeping thread before the designated sleep time is over. This can be achieved using the `Thread.interrupt()` method, which sends an interrupt signal to the thread, causing it to terminate its sleep and potentially enter a terminated or uncaught exception state. Understanding how to properly manage and wake up sleeping threads is crucial for efficient and error-free multithreaded programming in Java.
What You'll Learn
- Thread Synchronization: Use `synchronized` blocks or `ReentrantLock` to ensure only one thread accesses the resource at a time
- Interrupt Method: Call `Thread.interrupt()` to signal the thread to stop and wake it up
- Wait and Notify: Employ `Thread.sleep()` and `Object.wait()` to pause execution and wake up when conditions are met
- Thread Priority: Adjust thread priority to make it more likely to be scheduled for execution
- Thread Pooling: Utilize a thread pool to manage and reuse threads, ensuring efficient resource utilization
Thread Synchronization: Use `synchronized` blocks or `ReentrantLock` to ensure only one thread accesses the resource at a time
Thread synchronization is a crucial concept in Java to ensure that multiple threads do not simultaneously access shared resources, which can lead to data corruption or inconsistent behavior. One of the primary methods to achieve this is by using the `synchronized` keyword, which provides a simple and effective way to lock a block of code, ensuring that only one thread can execute it at a time. When a thread enters a `synchronized` block, it acquires the intrinsic lock on the object, and other threads attempting to access the same object will be blocked until the current thread releases the lock.
The `synchronized` keyword can be applied to methods or blocks of code within methods. For instance, if you have a method that modifies a shared data structure, you can make it `synchronized` to prevent concurrent access. Here's a basic example:
Java
Public class SharedResource {
Private int value = 0;
Public synchronized void increment() {
Value++;
}
Public int getValue() {
Return value;
}
}
In this example, the `increment` method is `synchronized`, ensuring that only one thread can increment the `value` at a time.
While `synchronized` blocks are easy to use, they come with some performance overhead due to the intrinsic locking mechanism. A more fine-grained approach is provided by the `ReentrantLock` class, which offers more control over locking and unlocking. `ReentrantLock` allows for nested locking, meaning a thread can acquire multiple locks in a specific order and release them in the reverse order. This is particularly useful when dealing with complex locking scenarios.
Here's how you can use `ReentrantLock`:
Java
Public class ReentrantLockExample {
Private ReentrantLock lock = new ReentrantLock();
Public void criticalSection() {
Lock.lock();
Try {
// Code that needs synchronization
} finally {
Lock.unlock();
}
}
}
In this code, the `lock` object is acquired before entering the critical section, and it is automatically released when the block is exited, regardless of whether an exception is thrown or not.
Both `synchronized` blocks and `ReentrantLock` provide powerful tools for managing thread synchronization. The choice between them depends on the specific requirements of your application. While `synchronized` is simpler and often sufficient for most cases, `ReentrantLock` offers more flexibility and can be beneficial when dealing with complex locking strategies or when performance is a critical factor.
The Power of Sleep: Why Waking Up Late is a Myth
You may want to see also
Interrupt Method: Call `Thread.interrupt()` to signal the thread to stop and wake it up
The `Thread.interrupt()` method is a powerful tool in Java for managing and waking up sleeping threads. When you call `interrupt()` on a thread, it sends a signal to that thread, indicating that it should stop its current task and potentially wake up from its sleep state. This method is particularly useful when you want to gracefully terminate a thread's execution or when you need to handle exceptions that might occur during the thread's operation.
Here's a step-by-step guide on how to use the `interrupt()` method to wake up a sleeping thread:
- Identify the Sleeping Thread: First, you need to determine which thread is in a sleeping state. This could be a thread that has called the `sleep()` method or is waiting for a specific condition to be met. You can use the `Thread.sleep()` method to put your thread to sleep for a specified duration or until a certain condition is fulfilled.
- Interrupt the Thread: Once you've identified the sleeping thread, you can call the `interrupt()` method on that specific thread instance. This action sets a flag on the thread, indicating that it should be interrupted. For example:
```java
Thread myThread = new Thread(new Runnable() {
@Override
Public void run() {
Try {
// Thread's sleep code
} catch (InterruptedException e) {
// Handle interruption
}
}
});
MyThread.interrupt();
```
- Handling Interruption: Inside the thread's `run()` method, it's essential to handle the `InterruptedException` that might be thrown when the thread is interrupted. This ensures that the thread can gracefully exit its current operation and respond to the interruption. You can use a try-catch block to catch this exception and perform any necessary cleanup or termination tasks.
- Checking for Interruption: After the thread's operation, it's good practice to check if the thread was interrupted using the `Thread.interrupted()` method. This method returns `true` if the thread has been interrupted and has not yet been reset. You can use this check to decide whether to proceed with the thread's original task or terminate it.
```java
If (Thread.interrupted()) {
// Handle interruption and cleanup
}
```
By utilizing the `interrupt()` method, you can effectively signal a sleeping thread to stop its current operation and wake up. This approach is especially useful when you need to manage thread lifecycles, handle exceptions, or coordinate the termination of multiple threads in a Java application. Remember to handle interruptions gracefully to ensure the stability and reliability of your program.
Revive Numb Hand: Tips for Tingling Relief and Awakening Sleep
You may want to see also
Wait and Notify: Employ `Thread.sleep()` and `Object.wait()` to pause execution and wake up when conditions are met
When working with multithreaded applications in Java, it's common to encounter situations where one thread needs to wait for another thread to complete a task or reach a certain state before proceeding. This is where the concepts of `Thread.sleep()` and `Object.wait()` come into play, providing mechanisms to pause the execution of a thread and be awakened when specific conditions are met.
`Thread.sleep()` is a static method of the `Thread` class that allows a thread to sleep for a specified amount of time. It is useful when you want a thread to temporarily pause its execution and resume after a certain duration. For example, if you have a thread responsible for reading data from a file, you might use `Thread.sleep()` to introduce a delay between reads to avoid overwhelming the file system. However, it's important to note that `Thread.sleep()` does not release the lock on any objects, so other threads might not be able to access shared resources during this time.
On the other hand, `Object.wait()` is a method of the `Object` class and is used in conjunction with the `synchronized` keyword to implement mutual exclusion and synchronization in Java. When a thread calls `Object.wait()`, it releases the lock on the object and goes to sleep until another thread calls `Object.notify()` or `Object.notifyAll()` on the same object. This mechanism is particularly useful when multiple threads need to access a shared resource, and you want to ensure that only one thread can access it at a time.
To effectively use `wait()` and `notify()`, you should follow these steps: First, identify the shared resource that needs to be synchronized. Then, use the `synchronized` keyword to ensure that only one thread can access this resource at a time. Inside the critical section, use `Object.wait()` to release the lock and pause the thread's execution when a certain condition is not met. When the condition is satisfied, call `Object.notify()` or `Object.notifyAll()` to wake up waiting threads and allow them to proceed.
It's important to note that using `wait()` and `notify()` requires careful synchronization to avoid deadlocks and race conditions. Properly managing the synchronization and ensuring that threads are awakened in the correct order is crucial for the correct functioning of your multithreaded application. By combining `Thread.sleep()` and `Object.wait()` with appropriate synchronization, you can effectively manage the execution flow of multiple threads and ensure that your Java application operates efficiently and reliably.
Revive Deep Sleep: Effective Strategies to Gently Awaken
You may want to see also
Thread Priority: Adjust thread priority to make it more likely to be scheduled for execution
In Java, thread scheduling is managed by the operating system, and it is crucial to understand how thread priorities can influence the execution order. Thread priority is a mechanism used by the Java Virtual Machine (JVM) to determine the relative importance of threads, with higher priority threads being given preference when the CPU is available. This concept is particularly useful when you want to ensure that certain threads are executed before or after others, especially when dealing with time-sensitive operations.
Each thread in Java has a priority value ranging from 1 (lowest) to 10 (highest). By default, threads are created with a priority of 5, which is the normal priority. However, you can adjust this priority to make a thread more likely to be scheduled for execution. For instance, if you have a critical task that needs to be completed immediately, you can set its priority to 10, ensuring it receives the highest possible preference. Conversely, for less time-critical tasks, a lower priority can be assigned.
To adjust thread priority, you can use the `setPriority()` method of the `Thread` class. This method takes an integer argument representing the new priority level. For example:
Java
Thread.setPriority(Thread.MIN_PRIORITY); // Minimum priority
Thread.setPriority(Thread.NORM_PRIORITY); // Normal priority (default)
Thread.setPriority(Thread.MAX_PRIORITY); // Maximum priority
It's important to note that while thread priority can influence scheduling, it does not guarantee execution order. The JVM's scheduler has its own criteria for deciding which thread to execute, and priority is just one of the factors considered. Other factors include thread state, available CPU time, and the fairness settings of the scheduler.
Additionally, setting a thread to the highest priority does not necessarily mean it will always run. The JVM may still choose to execute other threads with lower priorities, especially if they are in a ready state and have been waiting for a longer time. Therefore, while adjusting thread priority is a useful technique, it should be used judiciously, considering the overall system requirements and thread interactions.
Ode to a Nightingale: The Eternal Dilemma of Waking or Sleeping
You may want to see also
Thread Pooling: Utilize a thread pool to manage and reuse threads, ensuring efficient resource utilization
Thread pooling is a powerful technique in Java to manage and optimize the use of threads, ensuring efficient resource utilization and improved application performance. It involves creating a pool of pre-initialized threads that can be reused as needed, rather than creating and destroying threads dynamically for each task. This approach helps to reduce the overhead associated with thread creation and provides a more efficient way to handle concurrent operations.
When a task arrives, the thread pool assigns it to an available thread from the pool. If no threads are available, the task is added to a queue, and a new thread is created and added to the pool when a thread becomes available. This mechanism ensures that threads are utilized effectively, preventing the creation of an excessive number of threads that could lead to resource exhaustion. By reusing threads, thread pooling reduces the time and resources required to start and stop threads, resulting in improved responsiveness and better overall system performance.
The benefits of thread pooling are particularly evident in scenarios with a high volume of short-lived tasks. For example, in a web application, multiple user requests might arrive simultaneously, and each request could trigger multiple database queries or other I/O-bound operations. With thread pooling, these tasks can be efficiently managed, ensuring that the application can handle the load without overwhelming the system resources. Threads in the pool can be reused for subsequent tasks, reducing the time spent on thread creation and improving the application's ability to respond promptly to user requests.
Implementing a thread pool involves creating a thread pool manager that controls the pool's size, keeps track of available threads, and manages the task queue. The pool size can be configured to match the expected workload, ensuring that there are enough threads to handle the anticipated number of tasks without over-provisioning resources. Advanced thread pool implementations might include features like thread recycling, where threads are moved between pools based on their type of work, and thread timeout mechanisms to prevent idle threads from consuming resources indefinitely.
In summary, thread pooling is a valuable technique for managing threads and optimizing resource utilization in Java applications. By reusing threads from a pool, developers can ensure efficient handling of concurrent tasks, improve application responsiveness, and enhance overall system performance, especially in scenarios with a high volume of short-lived tasks. Understanding and implementing thread pooling can lead to more robust and scalable Java applications.
Revive Your Mind: Strategies to Jumpstart Your Brain After Sleepless Nights
You may want to see also
Frequently asked questions
In Java, you can use the `Thread.interrupt()` method to wake up a sleeping thread. When a thread is interrupted, it sets a flag that indicates the thread should terminate its execution. You can check the `Thread.isInterrupted()` method to determine if the thread has been interrupted.
`Thread.sleep()` is used to pause the execution of a thread for a specified amount of time, after which the thread resumes its execution. On the other hand, `Thread.interrupt()` is used to signal a thread to stop its execution and can be used to wake up a sleeping thread.
Yes, you can use `Thread.interrupt()` to wake up a thread that is blocked on an I/O operation. When a thread is interrupted while waiting for I/O, it will be unblocked and may continue its execution.
Calling `Thread.interrupt()` on a thread that is not sleeping will set the interrupted status of the thread. This means that any subsequent calls to `Thread.isInterrupted()` will return `true`, indicating that the thread has been interrupted. However, it won't immediately stop the thread's execution unless it is in a critical section or has a specific interrupt-handling mechanism in place.