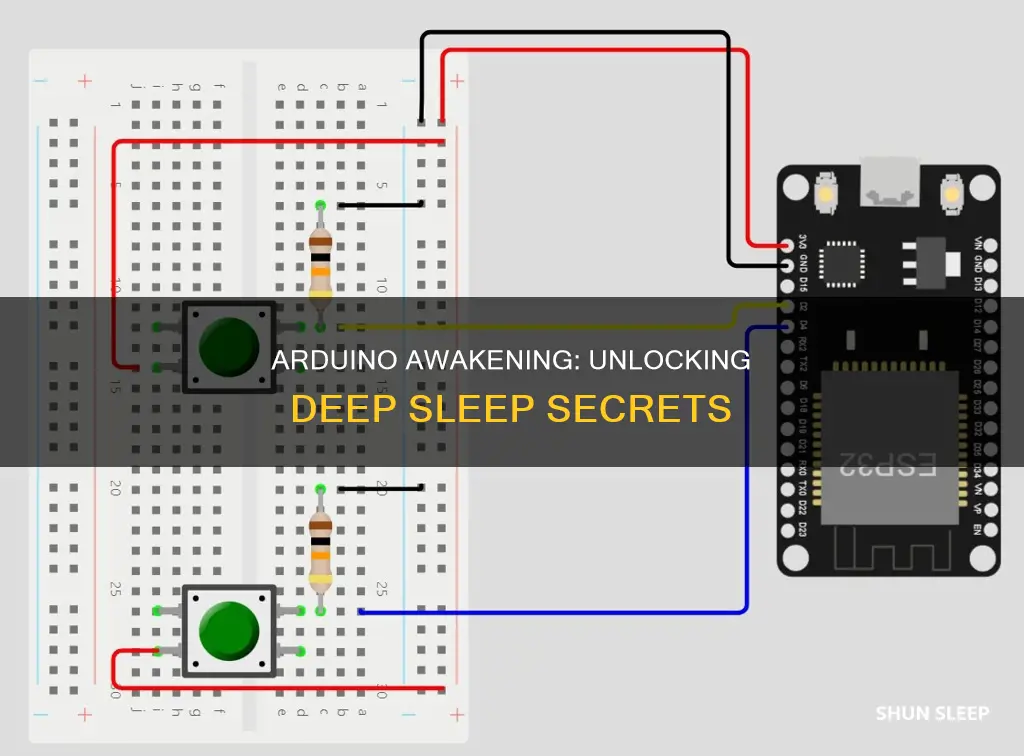
Understanding how to wake up an Arduino from sleep is crucial for optimizing power usage and ensuring your projects run efficiently. This guide will explore the various methods and techniques available to bring your Arduino board out of its low-power state, enabling it to resume its tasks and respond to external stimuli. By the end of this article, you'll have the knowledge to effectively manage your Arduino's power consumption and keep your projects running smoothly.
Characteristics | Values |
---|---|
Power-on Reset | Resets the Arduino upon power-up |
External Interrupts | Can wake the Arduino from sleep when triggered |
Timer Interrupts | Utilizes the Timer/Counter peripherals to wake the Arduino |
External Interrupts (Pin Change Interrupt) | Wakes the Arduino when a specific pin changes state |
Watchdog Timer | A dedicated timer that can be used to wake the Arduino |
Software Interrupts | Can be programmed to wake the Arduino from sleep |
External Clock | An external clock source can be used to wake the Arduino |
Pin Change Detection | Arduino pins can be configured to detect changes and wake the device |
Timer Compare Match | Compares the timer value with a predefined threshold to wake the Arduino |
External Interrupts (Interrupt-on-Change) | A specific pin can be configured to wake the Arduino on a change in its state |
What You'll Learn
- Understanding Arduino Sleep Modes: Learn about different sleep modes and their functions
- Setting Up Sleep Mode: Configure Arduino to enter sleep mode
- Using External Interrupts: Employ external interrupts to wake the Arduino
- Power Management: Optimize power consumption to extend sleep duration
- Software Interrupts: Utilize software interrupts to wake the Arduino from sleep
Understanding Arduino Sleep Modes: Learn about different sleep modes and their functions
The Arduino platform offers various sleep modes to conserve power and improve the efficiency of your projects. Understanding these modes is crucial for optimizing your Arduino's performance, especially in battery-powered applications. Here's an overview of the different sleep modes and their functions:
Deep Sleep Mode: This mode is a powerful feature that puts the Arduino into a very low-power state, consuming minimal current. In deep sleep, the Arduino's internal oscillator is disabled, and the CPU enters a low-power mode. This mode is ideal for applications where you need to save power for extended periods. During deep sleep, the Arduino can be woken up by an interrupt or an external signal, ensuring it can respond to events even when in a low-power state. This mode is particularly useful for projects that require long-term monitoring or data logging, as it allows the Arduino to remain in a deep sleep until an event occurs, thus conserving energy.
Power-Down Mode: In this mode, the Arduino's digital pins and analog pins are powered down, and the CPU enters a low-power state. The Arduino's internal oscillator remains active, allowing it to wake up quickly in response to interrupts. Power-down mode is useful when you want to save power but still need to be responsive to certain events. For example, you can use this mode to wake up the Arduino when a button is pressed or a sensor detects a specific condition. The CPU's low-power state ensures that the Arduino consumes minimal power while remaining ready to respond to external inputs.
Sleep Mode: This mode is a balance between power conservation and responsiveness. In sleep mode, the Arduino's internal oscillator is enabled, allowing it to wake up quickly in response to interrupts. During sleep, the Arduino's digital and analog pins are powered down, and the CPU enters a low-power state. This mode is suitable for applications where you want to save power while still being able to respond to specific events. For instance, you can use sleep mode to wake up the Arduino when a timer expires or when a specific pin state changes. The low-power state ensures that the Arduino remains in a ready-to-respond condition without consuming excessive power.
Understanding these sleep modes is essential for optimizing your Arduino's performance and power consumption. By choosing the appropriate sleep mode, you can ensure that your Arduino project operates efficiently, especially in battery-powered applications. Remember that the specific implementation details may vary depending on the Arduino board and the library functions used.
Wake Your Mac from Slumber: Remote Tips for Instant Action
You may want to see also
Setting Up Sleep Mode: Configure Arduino to enter sleep mode
To set up sleep mode on your Arduino, you need to understand the specific microcontroller you are using, as different models have varying capabilities and requirements. The Arduino platform offers several options for power-saving modes, which can be crucial for extending battery life or reducing power consumption in projects. Here's a step-by-step guide to configuring your Arduino to enter sleep mode:
- Choose the Right Sleep Mode: Arduino boards often have multiple sleep modes, each with different power-saving characteristics. Common sleep modes include 'Deep Sleep' and 'Light Sleep'. Deep Sleep is a low-power mode where the Arduino can be woken up by an external interrupt or a timer. Light Sleep, on the other hand, is a more aggressive power-saving mode but may require more specific wake-up conditions. Select the mode that best suits your project's needs.
- Modify the Arduino Sketch: Open the Arduino IDE and locate the sketch (program) you want to modify. You'll need to add specific code to enable sleep mode. Start by including the necessary libraries, such as the 'Sleep' library, which provides functions to manage sleep. Then, add the appropriate sleep function calls. For example, you can use the `delayMicroseconds()` function to put the Arduino into a low-power state. Here's a basic example:
Cpp
#include
Void setup() {
// Your setup code here
}
Void loop() {
// Your main loop code
DelayMicroseconds(1000000); // Example: Put the Arduino to sleep for 1 second
Sleep(1000); // Put the Arduino to sleep for 1000 milliseconds (1 second)
}
- Wake-Up Conditions: Define how your Arduino should wake up from sleep. This could be triggered by an external interrupt, a timer, or a specific pin change. For instance, you can set up an interrupt on a particular pin that wakes the Arduino when it detects a specific signal. The Arduino's documentation will guide you through the process of creating interrupts and setting wake-up conditions.
- Test and Adjust: After implementing the sleep mode, test your Arduino sketch thoroughly. Ensure that the sleep mode functions as expected and that the wake-up conditions work correctly. You may need to adjust the sleep duration or wake-up settings based on your project's requirements.
Remember, the specific code and libraries used may vary depending on your Arduino model and the microcontroller's manufacturer. Always refer to the official Arduino documentation and the microcontroller's datasheet for detailed instructions tailored to your hardware.
Mastering Early Bird Hours: Tips for a Productive Morning Routine
You may want to see also
Using External Interrupts: Employ external interrupts to wake the Arduino
When it comes to waking up an Arduino from its sleep mode, using external interrupts is a powerful and efficient approach. This method allows you to trigger the Arduino's wake-up process in response to specific events or signals from the outside world. Here's a detailed guide on how to utilize external interrupts for this purpose:
Understanding External Interrupts:
External interrupts are signals generated by external devices or sensors connected to the Arduino. These interrupts can be used to notify the Arduino of an event, prompting it to exit sleep mode and execute specific code. The Arduino has dedicated pins for external interrupts, typically labeled as 'Interrupt Pins' or 'Interrupt 0' and 'Interrupt 1'. These pins can be configured to trigger an interrupt request when a specific condition is met.
Setting Up the External Interrupt:
To employ external interrupts for waking up the Arduino, you need to follow these steps:
- Identify the external device or sensor that will generate the interrupt signal. This could be a button, a light sensor, a vibration sensor, or any other device that can provide a physical or digital input.
- Connect the output of the external device to one of the interrupt pins on the Arduino. Ensure that the connection is clean and secure to avoid any signal degradation.
- Use the Arduino's built-in interrupt function, `attachInterrupt()`, to associate the interrupt pin with a specific interrupt service routine (ISR). This routine will contain the code that the Arduino will execute when the interrupt is triggered.
Writing the Interrupt Service Routine (ISR):
The ISR is a critical part of the process, as it defines the actions the Arduino should take when the external interrupt occurs. Here's a basic structure:
- Inside the ISR, you can add code to perform the desired action upon waking the Arduino. For example, you might want to read sensor data, update a display, or initiate a specific process.
- It is essential to use the `noInterrupts()` function to disable interrupts temporarily while executing the ISR to prevent multiple interrupts from occurring simultaneously.
- After the required actions, re-enable interrupts with `enableInterrupts()`.
Example Code:
Here's a simplified example to illustrate the concept:
Cpp
Void setup() {
PinMode(2, INPUT_PULLUP); // Set the interrupt pin as input with internal pull-up resistor
AttachInterrupt(digitalPinToInterrupt(2), myInterrupt, FALLING); // Configure interrupt on pin 2, triggered on falling edge
}
Void loop() {
// Arduino code can go here, but it will not execute while the interrupt is active
}
Void myInterrupt() {
// Code executed when the interrupt is triggered
// Example: read sensor data, update variables, etc.
// ...
// Re-enable interrupts after necessary actions
EnableInterrupts();
}
In this example, the Arduino is set to wake up when the interrupt pin 2 detects a falling edge (e.g., a button press). The ISR, `myInterrupt`, will then execute the required code.
By utilizing external interrupts, you can create a flexible and responsive Arduino system that can wake up and perform specific tasks in response to external events, making it a valuable technique for various projects and applications.
Uncover the Sleep/Wake Slider: A Guide to iPad Settings
You may want to see also
Power Management: Optimize power consumption to extend sleep duration
Power management is a critical aspect of optimizing the performance and longevity of Arduino projects, especially when aiming to extend sleep durations. The Arduino platform offers various power-saving modes and techniques to ensure your microcontroller remains in a low-power state for extended periods without sacrificing functionality. Here's a detailed guide on how to optimize power consumption and maximize sleep duration:
- Utilize Sleep Modes: Arduino boards often feature built-in sleep modes, which are essential for power conservation. These modes allow the microcontroller to enter a low-power state while retaining certain registers and variables. The most common sleep modes include Deep Sleep and Power-Down modes. Deep Sleep is ideal for applications where you need to maintain a specific time or interrupt-based functionality while saving power. Power-Down mode is even more aggressive, cutting off most power to the microcontroller, making it suitable for situations where you need to minimize power consumption to the absolute minimum.
- Enable Brownout Reset (BOR): The Brownout Reset feature is a safety mechanism that can be enabled in the Arduino's power management settings. It monitors the voltage on the VCC pin and resets the microcontroller if the voltage drops below a certain threshold. While it might seem counterintuitive for power management, BOR is crucial for preventing damage to the microcontroller during voltage fluctuations. By setting an appropriate BOR threshold, you can ensure that your Arduino remains in a stable state, even during power interruptions, thus maintaining the integrity of your sleep cycles.
- Optimize Digital and Analog Pins: The way you use digital and analog pins can significantly impact power consumption. When not in use, digital pins should be set to HIGH or LOW to avoid pulling the pin's internal pull-up or pull-down resistors. Similarly, analog pins should be configured to a high impedance state when not reading. These simple steps can prevent unnecessary current draw and contribute to longer sleep durations.
- Use External Interrupts: External interrupts can be triggered by external events, allowing your Arduino to wake up from sleep without consuming power in the main loop. This is particularly useful for time-critical tasks or when you need to respond to specific events. By utilizing external interrupts, you can minimize the time the microcontroller spends in an active state, thereby extending the overall sleep duration.
- Implement Power-Saving Loops: Consider creating custom power-saving loops that are executed during sleep. These loops can perform minimal tasks, such as reading sensors or checking for specific conditions, without fully waking up the microcontroller. By keeping the system in a low-power state, you can significantly reduce power consumption while still maintaining the ability to respond to critical events.
- External Power Sources: For projects requiring extended operation, consider using external power sources like solar panels or batteries. This approach allows you to power the Arduino without relying solely on the USB connection or an AC adapter, which can be less reliable for long-term projects. Proper voltage regulation and management are essential to ensure the Arduino operates within the optimal voltage range during sleep and active states.
By implementing these power management techniques, you can significantly extend the sleep duration of your Arduino projects while maintaining functionality. It's essential to strike a balance between power conservation and the responsiveness required for your specific application. Regularly testing and optimizing your code will ensure that your Arduino operates efficiently and reliably in both sleep and active modes.
Crystal Energy: Awakening the Slumberer with Vibrant Power
You may want to see also
Software Interrupts: Utilize software interrupts to wake the Arduino from sleep
When working with the Arduino, understanding how to wake it from a sleep state is crucial for optimizing power consumption and ensuring timely responses. One effective method to achieve this is by utilizing software interrupts. This approach leverages the Arduino's interrupt system to wake the microcontroller from its low-power mode.
Software interrupts are a powerful tool in the Arduino's arsenal, allowing you to define custom interrupt service routines (ISRs) that can be triggered by specific events or conditions. By programming a software interrupt, you can wake the Arduino from sleep and execute a dedicated function to handle the interrupt. This is particularly useful when you need to perform time-critical tasks or respond to external events while the board is in a low-power state.
To implement this, you'll need to use the `attachInterrupt()` function provided by the Arduino framework. This function allows you to associate an interrupt with a specific pin or event. When the interrupt is triggered, the associated interrupt service routine (ISR) is executed, providing a way to wake the Arduino and perform necessary actions. For example, you can set up an interrupt on a digital pin that changes state, indicating an external event, and use this interrupt to wake the board and process the event.
Here's a simplified example to illustrate the concept:
Cpp
Void setup() {
// Initialize the pin as an interrupt input
PinMode(2, INPUT_PULLUP);
// Attach the interrupt handler function
AttachInterrupt(digitalPinToInterrupt(2), myInterruptHandler, FALLING);
}
Void loop() {
// Your main loop code here
// ...
}
Void myInterruptHandler() {
// Interrupt service routine
// Wake the Arduino from sleep and process the event
// ...
}
In this example, the `attachInterrupt()` function is used to set up an interrupt on pin 2, which is configured as an input with a pull-up resistor. When the signal on pin 2 transitions from high to low (FALLING edge), the `myInterruptHandler` function is executed, allowing you to wake the Arduino and perform specific actions.
By utilizing software interrupts, you can efficiently manage power consumption while still being able to respond to external events or time-sensitive tasks. This method provides a flexible and powerful way to wake the Arduino from sleep, ensuring your projects can operate efficiently and react to various stimuli.
Fetal Sleep Patterns: Exploring the Early Stages of Sleep-Wake Rhythms
You may want to see also
Frequently asked questions
To wake up an Arduino board from its sleep mode, you can use the built-in functions provided by the Arduino framework. The `delay()` function can be utilized to create a delay, which will wake up the board. For example, you can use `delay(1000)` to introduce a 1-second delay, allowing the board to wake up.
Yes, you can utilize specific pins or interrupts to wake up the Arduino. For instance, you can configure a pin as an interrupt and set a specific condition to trigger the wake-up. When the interrupt condition is met, the Arduino will exit sleep mode and start executing the code. This method provides a more customized way to wake up the board based on specific events.
Absolutely! External interrupts or sensors can be employed to wake up the Arduino and initiate specific actions. You can connect a sensor to a pin and set it as an interrupt. When the sensor detects a change, the interrupt will be triggered, causing the Arduino to wake up and execute the associated code. This approach is useful for creating interactive systems where the Arduino responds to external events.